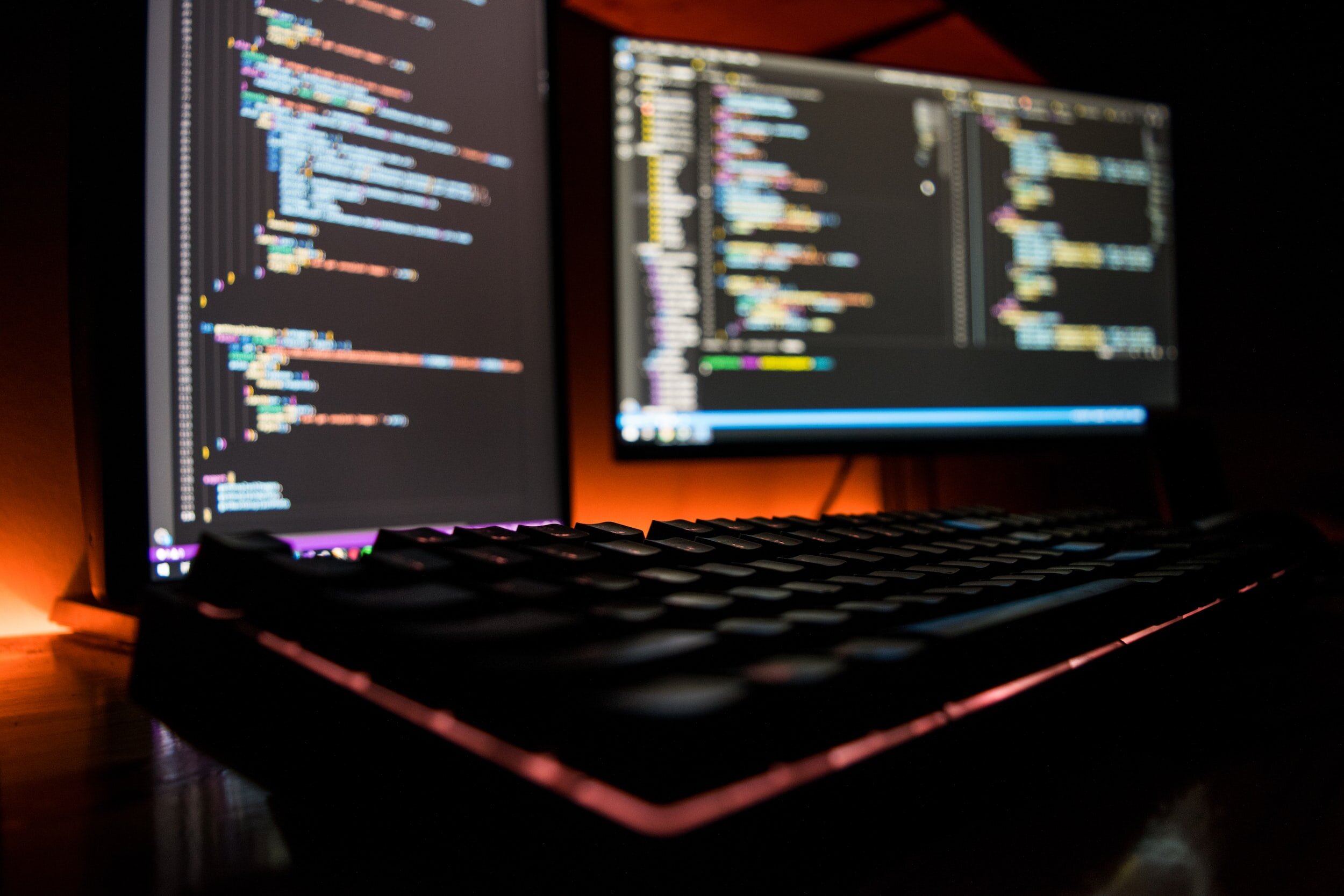
IT COURSES
Programming Language
Certified Training Course.
Programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms.
COURSE OUTLINE
PHP
Lesson 1: PHP – The Basics
HTML Embedding
Comments
Variables
The Data Types
Constants
Operators
Lesson 2: The Control Structures
Conditional Structures
The Loop Structures
The Code Inclusion Structures
Lesson 3: The Functions of PHP
The User-Defined Functions
The Scope of a Function
The “By Value” Method of Returning a Value
The “By Reference” Method of Returning a Value
How to Declare a Function Parameter
The Static Variables
Lesson 4: Object-Oriented Programming
Objects – The Basics
Class Declarations
Creating Class Instances
The Destructor Functions
How to Use “$this”
The Class Constants
Cloning an Object
Polymorphism
Lesson 5: How to Handle Exceptions
Errors
Failures
Program bug
Lesson 6: The Advanced Concepts of Object-Oriented Programming
The Overloading Capabilities of OOP
The Iterators
The Design Patterns
Lesson 7: Using PHP to Create an Application
PHP and HTML
Users’ Input
How to Handle User Input Safely
Sessions & File Uploads
Architecture
Lesson 8: Databases and the PHP Language
MySQL – The Basics
More Information about MySQL
Database Queries
HTML
Getting Started
Be Aware of the Current Version of HTML
Identify the Best HTML Editor for You
Document Setup & Style Sheet Setup
Create an HTML File
Preview an HTML File in a Browser
Set Up Style Sheets in an HTML File
New and Notable Color Options
Working with Text
Markup Text
Style Text
Page Structure
Organize Sections of Content
Organize Text
Positioning Page Elements
Uses of Style Sheets for Page Layout
Multicolumn Fluid Page Layout
CSS Page Layouts
Adding the Content & Links
Links to other web pages
Within the same web page
Effective Links & Style links
Working with Images
Web Image Sources
Graphics Software
Working with Multimedia
Plug-ins
Link & Embed
Creating Lists
Ordered, Unordered & Definition
Styles
Using Tables
Table Structure
Formatting
Creating Forms
Basic Form
Validate the content
Process
Beyond Static HTML
JavaScript and HTML5 APIs
Sample Scripts
Publishing Pages
Domain
Hosting
Public Debut
Host Computer
HTML for Email
Email Standard Project
HTML Email
Don’t send Spam
CSS Support in Email Clients
JAVA
Data Types, Variables and Arrays
Data Types
Variables
Arrays
Operators
Assignment Operator
Arithmetic Operators
Relational Operators
Bitwise Operators
Control Structures
Java Selection Statements
Iteration Statements
Jump Statements
Fundamentals of Classes & Objects
Class
Declaring Objects
Using Methods
Polymorphism
Static Keyword
Access Control
Private Constructors
Inheritance
Introduction
Inheritance and Member Access
Super Keyword
Types of Inheritance
Method Overriding
Packages and Interfaces
Defining a Package
Accessing Package Members
Compiling and Executing a Package
Access Control in Packages
Interfaces
Exception Handling
Exception Handling Mechanism
Life without Exception Handling
Types of Exceptions
Multithreading
Single Threaded vs Multithreaded Model
Thread Class and Runnable Interface
Life Cycle of a Thread
Java Libraries
String Handling
Wrapper Classes
Boxing and Unboxing
Applets and AWT
Applet Class and Its Methods
The Applet Tag
Life Cycle of Applets
Creating and Running an Applet
Event Handling
Event Delegation Model
Using Event Delegation Model
Adapter Class
Nested Classes
Networking
Networking Basics
Networking Classes
Sockets
Datagrams
JDBC
Servlets
SQL
Part I: Basic Concepts.
Lesson 1: Relational Database Fundamentals.
Lesson 2: SQL Fundamentals.
Lesson 3: The Components of SQL.
Part II: Using SQL to Build Databases.
Lesson 4: Building and Maintaining a Simple Database Structure.
Lesson 5: Building a Multi-table Relational Database.
Part III: Storing and Retrieving Data.
Lesson 6: Manipulating Database Data.
Lesson 7: Specifying Values.
Lesson 8: Using Advanced SQL Value Expressions.
Lesson 9: Zeroing In on the Data You Want.
Lesson 10: Using Relational Operators.
Lesson 11: Delving Deep with Nested Queries.
Lesson 12: Recursive Queries.
Part IV: Controlling Operations.
Lesson 13: Providing Database Security.
Lesson 14: Protecting Data.
Lesson 15: Using SQL within Applications.
Part V: Taking SQL to the Real World.
Lesson 16: Accessing Data with ODBC and JDBC.
Lesson 17: Operating on XML Data with SQL.
Part VI: Advanced Topics.
Lesson 18: Stepping through a Dataset with Cursors.
Lesson 19: Adding Procedural Capabilities with Persistent Stored Modules
Lesson 20: Handling Errors.
Lesson 21: Triggers.
VB.NET
Part I: Creating a Visual Basic .NET Program
Lesson 1: How Visual Basic .NET Works
Lesson 2: Using the Visual Basic .NET User Interface
Lesson 3: Designing Your First User Interface
Lesson 4: Writing BASIC Code
Part II: Creating User Interfaces
Lesson 5: User Interface Design
Lesson 6: Designing Forms
Lesson 7: Boxes and Buttons for Making Choices
Lesson 8: Text Boxes and Labels for Typing and Showing Words
Lesson 9: Showing Choices with List and Combo Boxes
Lesson 10: Fine-Tuning the Appearance of Your User Interface
Part III: Making Menus
Lesson 11: Creating and Editing Pull-Down Menus
Lesson 12: Submenus, Growing Menus, and Pop-Up Menus
Lesson 13: Showing Dialog Boxes
Part IV: The Basics of Writing Code
Lesson 14: Writing Event-Handling Procedures
Lesson 15: Using Variables
Lesson 16: Getting Data from the User
Lesson 17: Math 101: Arithmetic, Logical, and Comparison Operators
Lesson 18: Strings and Things
Lesson 19: Defining Constants and Using Comments
Lesson 20: Storing Stuff in Data Structures
Lesson 21: Killing Bugs
Part V: Making Decisions and Getting Loopy
Lesson 22: Making Decisions with If-Then Statements
Lesson 23: The Select Case Statement
Lesson 24: Repeating Yourself with Loops
Lesson 25: For-Next Loops That Can Count
Lesson 26: Nested Loops and Quick Exits
Part VI: Writing Subprograms
Lesson 27: General Procedures (Subprograms That Everyone Can Share)
Lesson 28: Passing Arguments
Lesson 29: Function, a Unique Type of Subprogram
Part VII: Understanding Object-Oriented Programming
Lesson 30: What the Heck Is Object-Oriented Programming?
Lesson 31: Getting Some Class with Object-Oriented Programming
Lesson 32: Using Inheritance and Overloading
View more information.
-
Enroll & become certified, Enhance your career with the certification and your learnings.
-
Our courses are flexible with no time plan.
-
Our classes are available online or face-to-face. with flexible timing scheduling.
-
You must complete the training & Exam to achieve the certification.
-
To get a quote on our courses, you can contact us on 8004852